mirror of
https://github.com/xzeldon/htop.git
synced 2024-12-25 15:25:45 +00:00
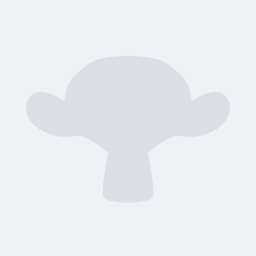
According to the Linux kernel documentation, "SwapCached" tracks "memory that once was swapped out, is swapped back in but still also is in the swapfile (if memory is needed it doesn't need to be swapped out AGAIN because it is already in the swapfile. This saves I/O)."
261 lines
7.0 KiB
C
261 lines
7.0 KiB
C
/*
|
|
htop - dragonflybsd/Platform.c
|
|
(C) 2014 Hisham H. Muhammad
|
|
(C) 2017 Diederik de Groot
|
|
Released under the GNU GPLv2, see the COPYING file
|
|
in the source distribution for its full text.
|
|
*/
|
|
|
|
#include "Platform.h"
|
|
#include "Macros.h"
|
|
#include "Meter.h"
|
|
#include "CPUMeter.h"
|
|
#include "MemoryMeter.h"
|
|
#include "SwapMeter.h"
|
|
#include "TasksMeter.h"
|
|
#include "LoadAverageMeter.h"
|
|
#include "UptimeMeter.h"
|
|
#include "ClockMeter.h"
|
|
#include "DateMeter.h"
|
|
#include "DateTimeMeter.h"
|
|
#include "HostnameMeter.h"
|
|
#include "DragonFlyBSDProcess.h"
|
|
#include "DragonFlyBSDProcessList.h"
|
|
|
|
#include <sys/types.h>
|
|
#include <sys/sysctl.h>
|
|
#include <sys/time.h>
|
|
#include <sys/resource.h>
|
|
#include <vm/vm_param.h>
|
|
#include <time.h>
|
|
#include <math.h>
|
|
|
|
|
|
const ProcessField Platform_defaultFields[] = { PID, USER, PRIORITY, NICE, M_VIRT, M_RESIDENT, STATE, PERCENT_CPU, PERCENT_MEM, TIME, COMM, 0 };
|
|
|
|
const SignalItem Platform_signals[] = {
|
|
{ .name = " 0 Cancel", .number = 0 },
|
|
{ .name = " 1 SIGHUP", .number = 1 },
|
|
{ .name = " 2 SIGINT", .number = 2 },
|
|
{ .name = " 3 SIGQUIT", .number = 3 },
|
|
{ .name = " 4 SIGILL", .number = 4 },
|
|
{ .name = " 5 SIGTRAP", .number = 5 },
|
|
{ .name = " 6 SIGABRT", .number = 6 },
|
|
{ .name = " 7 SIGEMT", .number = 7 },
|
|
{ .name = " 8 SIGFPE", .number = 8 },
|
|
{ .name = " 9 SIGKILL", .number = 9 },
|
|
{ .name = "10 SIGBUS", .number = 10 },
|
|
{ .name = "11 SIGSEGV", .number = 11 },
|
|
{ .name = "12 SIGSYS", .number = 12 },
|
|
{ .name = "13 SIGPIPE", .number = 13 },
|
|
{ .name = "14 SIGALRM", .number = 14 },
|
|
{ .name = "15 SIGTERM", .number = 15 },
|
|
{ .name = "16 SIGURG", .number = 16 },
|
|
{ .name = "17 SIGSTOP", .number = 17 },
|
|
{ .name = "18 SIGTSTP", .number = 18 },
|
|
{ .name = "19 SIGCONT", .number = 19 },
|
|
{ .name = "20 SIGCHLD", .number = 20 },
|
|
{ .name = "21 SIGTTIN", .number = 21 },
|
|
{ .name = "22 SIGTTOU", .number = 22 },
|
|
{ .name = "23 SIGIO", .number = 23 },
|
|
{ .name = "24 SIGXCPU", .number = 24 },
|
|
{ .name = "25 SIGXFSZ", .number = 25 },
|
|
{ .name = "26 SIGVTALRM", .number = 26 },
|
|
{ .name = "27 SIGPROF", .number = 27 },
|
|
{ .name = "28 SIGWINCH", .number = 28 },
|
|
{ .name = "29 SIGINFO", .number = 29 },
|
|
{ .name = "30 SIGUSR1", .number = 30 },
|
|
{ .name = "31 SIGUSR2", .number = 31 },
|
|
{ .name = "32 SIGTHR", .number = 32 },
|
|
{ .name = "33 SIGLIBRT", .number = 33 },
|
|
};
|
|
|
|
const unsigned int Platform_numberOfSignals = ARRAYSIZE(Platform_signals);
|
|
|
|
const MeterClass* const Platform_meterTypes[] = {
|
|
&CPUMeter_class,
|
|
&ClockMeter_class,
|
|
&DateMeter_class,
|
|
&DateTimeMeter_class,
|
|
&LoadAverageMeter_class,
|
|
&LoadMeter_class,
|
|
&MemoryMeter_class,
|
|
&SwapMeter_class,
|
|
&TasksMeter_class,
|
|
&UptimeMeter_class,
|
|
&BatteryMeter_class,
|
|
&HostnameMeter_class,
|
|
&AllCPUsMeter_class,
|
|
&AllCPUs2Meter_class,
|
|
&AllCPUs4Meter_class,
|
|
&AllCPUs8Meter_class,
|
|
&LeftCPUsMeter_class,
|
|
&RightCPUsMeter_class,
|
|
&LeftCPUs2Meter_class,
|
|
&RightCPUs2Meter_class,
|
|
&LeftCPUs4Meter_class,
|
|
&RightCPUs4Meter_class,
|
|
&LeftCPUs8Meter_class,
|
|
&RightCPUs8Meter_class,
|
|
&BlankMeter_class,
|
|
NULL
|
|
};
|
|
|
|
void Platform_init(void) {
|
|
/* no platform-specific setup needed */
|
|
}
|
|
|
|
void Platform_done(void) {
|
|
/* no platform-specific cleanup needed */
|
|
}
|
|
|
|
void Platform_setBindings(Htop_Action* keys) {
|
|
/* no platform-specific key bindings */
|
|
(void) keys;
|
|
}
|
|
|
|
int Platform_getUptime() {
|
|
struct timeval bootTime, currTime;
|
|
int mib[2] = { CTL_KERN, KERN_BOOTTIME };
|
|
size_t size = sizeof(bootTime);
|
|
|
|
int err = sysctl(mib, 2, &bootTime, &size, NULL, 0);
|
|
if (err) {
|
|
return -1;
|
|
}
|
|
gettimeofday(&currTime, NULL);
|
|
|
|
return (int) difftime(currTime.tv_sec, bootTime.tv_sec);
|
|
}
|
|
|
|
void Platform_getLoadAverage(double* one, double* five, double* fifteen) {
|
|
struct loadavg loadAverage;
|
|
int mib[2] = { CTL_VM, VM_LOADAVG };
|
|
size_t size = sizeof(loadAverage);
|
|
|
|
int err = sysctl(mib, 2, &loadAverage, &size, NULL, 0);
|
|
if (err) {
|
|
*one = 0;
|
|
*five = 0;
|
|
*fifteen = 0;
|
|
return;
|
|
}
|
|
*one = (double) loadAverage.ldavg[0] / loadAverage.fscale;
|
|
*five = (double) loadAverage.ldavg[1] / loadAverage.fscale;
|
|
*fifteen = (double) loadAverage.ldavg[2] / loadAverage.fscale;
|
|
}
|
|
|
|
int Platform_getMaxPid() {
|
|
int maxPid;
|
|
size_t size = sizeof(maxPid);
|
|
int err = sysctlbyname("kern.pid_max", &maxPid, &size, NULL, 0);
|
|
if (err) {
|
|
return 999999;
|
|
}
|
|
return maxPid;
|
|
}
|
|
|
|
double Platform_setCPUValues(Meter* this, int cpu) {
|
|
const DragonFlyBSDProcessList* fpl = (const DragonFlyBSDProcessList*) this->pl;
|
|
int cpus = this->pl->cpuCount;
|
|
const CPUData* cpuData;
|
|
|
|
if (cpus == 1) {
|
|
// single CPU box has everything in fpl->cpus[0]
|
|
cpuData = &(fpl->cpus[0]);
|
|
} else {
|
|
cpuData = &(fpl->cpus[cpu]);
|
|
}
|
|
|
|
double percent;
|
|
double* v = this->values;
|
|
|
|
v[CPU_METER_NICE] = cpuData->nicePercent;
|
|
v[CPU_METER_NORMAL] = cpuData->userPercent;
|
|
if (this->pl->settings->detailedCPUTime) {
|
|
v[CPU_METER_KERNEL] = cpuData->systemPercent;
|
|
v[CPU_METER_IRQ] = cpuData->irqPercent;
|
|
this->curItems = 4;
|
|
percent = v[0] + v[1] + v[2] + v[3];
|
|
} else {
|
|
v[2] = cpuData->systemAllPercent;
|
|
this->curItems = 3;
|
|
percent = v[0] + v[1] + v[2];
|
|
}
|
|
|
|
percent = isnan(percent) ? 0.0 : CLAMP(percent, 0.0, 100.0);
|
|
|
|
v[CPU_METER_FREQUENCY] = NAN;
|
|
v[CPU_METER_TEMPERATURE] = NAN;
|
|
|
|
return percent;
|
|
}
|
|
|
|
void Platform_setMemoryValues(Meter* this) {
|
|
// TODO
|
|
const ProcessList* pl = this->pl;
|
|
|
|
this->total = pl->totalMem;
|
|
this->values[0] = pl->usedMem;
|
|
this->values[1] = pl->buffersMem;
|
|
this->values[2] = pl->cachedMem;
|
|
}
|
|
|
|
void Platform_setSwapValues(Meter* this) {
|
|
const ProcessList* pl = this->pl;
|
|
this->total = pl->totalSwap;
|
|
this->values[0] = pl->usedSwap;
|
|
this->values[1] = NAN;
|
|
}
|
|
|
|
char* Platform_getProcessEnv(pid_t pid) {
|
|
// TODO
|
|
(void)pid; // prevent unused warning
|
|
return NULL;
|
|
}
|
|
|
|
char* Platform_getInodeFilename(pid_t pid, ino_t inode) {
|
|
(void)pid;
|
|
(void)inode;
|
|
return NULL;
|
|
}
|
|
|
|
FileLocks_ProcessData* Platform_getProcessLocks(pid_t pid) {
|
|
(void)pid;
|
|
return NULL;
|
|
}
|
|
|
|
bool Platform_getDiskIO(DiskIOData* data) {
|
|
// TODO
|
|
(void)data;
|
|
return false;
|
|
}
|
|
|
|
bool Platform_getNetworkIO(unsigned long int* bytesReceived,
|
|
unsigned long int* packetsReceived,
|
|
unsigned long int* bytesTransmitted,
|
|
unsigned long int* packetsTransmitted) {
|
|
// TODO
|
|
*bytesReceived = 0;
|
|
*packetsReceived = 0;
|
|
*bytesTransmitted = 0;
|
|
*packetsTransmitted = 0;
|
|
return false;
|
|
}
|
|
|
|
void Platform_getBattery(double* percent, ACPresence* isOnAC) {
|
|
int life;
|
|
size_t life_len = sizeof(life);
|
|
if (sysctlbyname("hw.acpi.battery.life", &life, &life_len, NULL, 0) == -1)
|
|
*percent = NAN;
|
|
else
|
|
*percent = life;
|
|
|
|
int acline;
|
|
size_t acline_len = sizeof(acline);
|
|
if (sysctlbyname("hw.acpi.acline", &acline, &acline_len, NULL, 0) == -1)
|
|
*isOnAC = AC_ERROR;
|
|
else
|
|
*isOnAC = acline == 0 ? AC_ABSENT : AC_PRESENT;
|
|
}
|