mirror of
https://github.com/xzeldon/htop.git
synced 2025-01-31 09:07:25 +03:00
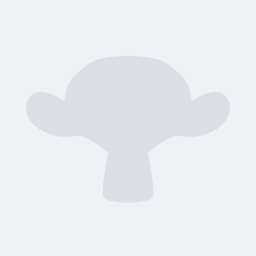
RichString_writeFrom takes a top spot during performance analysis due to the calls to mbstowcs() and iswprint(). Most of the time we know in advance that we are only going to print regular ASCII characters.
71 lines
1.7 KiB
C
71 lines
1.7 KiB
C
/*
|
|
htop - ListItem.c
|
|
(C) 2004-2011 Hisham H. Muhammad
|
|
Released under the GNU GPLv2, see the COPYING file
|
|
in the source distribution for its full text.
|
|
*/
|
|
|
|
#include "config.h" // IWYU pragma: keep
|
|
|
|
#include "ListItem.h"
|
|
|
|
#include <assert.h>
|
|
#include <stdlib.h>
|
|
#include <string.h>
|
|
|
|
#include "CRT.h"
|
|
#include "RichString.h"
|
|
#include "XUtils.h"
|
|
|
|
|
|
static void ListItem_delete(Object* cast) {
|
|
ListItem* this = (ListItem*)cast;
|
|
free(this->value);
|
|
free(this);
|
|
}
|
|
|
|
static void ListItem_display(const Object* cast, RichString* out) {
|
|
const ListItem* const this = (const ListItem*)cast;
|
|
assert (this != NULL);
|
|
|
|
if (this->moving) {
|
|
RichString_writeWide(out, CRT_colors[DEFAULT_COLOR],
|
|
#ifdef HAVE_LIBNCURSESW
|
|
CRT_utf8 ? "↕ " :
|
|
#endif
|
|
"+ ");
|
|
} else {
|
|
RichString_prune(out);
|
|
}
|
|
RichString_appendWide(out, CRT_colors[DEFAULT_COLOR], this->value);
|
|
}
|
|
|
|
ListItem* ListItem_new(const char* value, int key) {
|
|
ListItem* this = AllocThis(ListItem);
|
|
this->value = xStrdup(value);
|
|
this->key = key;
|
|
this->moving = false;
|
|
return this;
|
|
}
|
|
|
|
void ListItem_append(ListItem* this, const char* text) {
|
|
size_t oldLen = strlen(this->value);
|
|
size_t textLen = strlen(text);
|
|
size_t newLen = oldLen + textLen;
|
|
this->value = xRealloc(this->value, newLen + 1);
|
|
memcpy(this->value + oldLen, text, textLen);
|
|
this->value[newLen] = '\0';
|
|
}
|
|
|
|
static long ListItem_compare(const void* cast1, const void* cast2) {
|
|
const ListItem* obj1 = (const ListItem*) cast1;
|
|
const ListItem* obj2 = (const ListItem*) cast2;
|
|
return strcmp(obj1->value, obj2->value);
|
|
}
|
|
|
|
const ObjectClass ListItem_class = {
|
|
.display = ListItem_display,
|
|
.delete = ListItem_delete,
|
|
.compare = ListItem_compare
|
|
};
|