mirror of
https://github.com/xzeldon/htop.git
synced 2025-01-31 09:07:25 +03:00
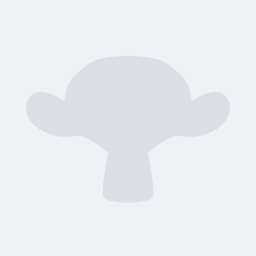
Implements the suggestion from https://github.com/htop-dev/htop/issues/399#issuecomment-747861013 Thanks to the refactors from 0bd5c8fb5da and 6393baa74e5, this was really easy and clean to do. It maintains the "Tree view always by PID" option in the Settings, which results in some specific behaviors such as "clicking on the column header to exit tree view" and "picking a new sort order to exit tree view", for the sake of the muscle memory of long time htop users. :)
97 lines
2.1 KiB
C
97 lines
2.1 KiB
C
#ifndef HEADER_Settings
|
|
#define HEADER_Settings
|
|
/*
|
|
htop - Settings.h
|
|
(C) 2004-2011 Hisham H. Muhammad
|
|
Released under the GNU GPLv2, see the COPYING file
|
|
in the source distribution for its full text.
|
|
*/
|
|
|
|
#include "config.h" // IWYU pragma: keep
|
|
|
|
#include <stdbool.h>
|
|
#include <stdint.h>
|
|
|
|
#include "Process.h"
|
|
|
|
|
|
#define DEFAULT_DELAY 15
|
|
|
|
typedef struct {
|
|
int len;
|
|
char** names;
|
|
int* modes;
|
|
} MeterColumnSettings;
|
|
|
|
typedef struct Settings_ {
|
|
char* filename;
|
|
MeterColumnSettings columns[2];
|
|
|
|
ProcessField* fields;
|
|
uint32_t flags;
|
|
int colorScheme;
|
|
int delay;
|
|
|
|
int direction;
|
|
int treeDirection;
|
|
ProcessField sortKey;
|
|
ProcessField treeSortKey;
|
|
|
|
bool countCPUsFromOne;
|
|
bool detailedCPUTime;
|
|
bool showCPUUsage;
|
|
bool showCPUFrequency;
|
|
#ifdef HAVE_SENSORS_SENSORS_H
|
|
bool showCPUTemperature;
|
|
bool degreeFahrenheit;
|
|
#endif
|
|
bool treeView;
|
|
bool treeViewAlwaysByPID;
|
|
bool showProgramPath;
|
|
bool shadowOtherUsers;
|
|
bool showThreadNames;
|
|
bool hideKernelThreads;
|
|
bool hideUserlandThreads;
|
|
bool highlightBaseName;
|
|
bool highlightMegabytes;
|
|
bool highlightThreads;
|
|
bool highlightChanges;
|
|
int highlightDelaySecs;
|
|
bool findCommInCmdline;
|
|
bool stripExeFromCmdline;
|
|
bool showMergedCommand;
|
|
bool updateProcessNames;
|
|
bool accountGuestInCPUMeter;
|
|
bool headerMargin;
|
|
bool enableMouse;
|
|
#ifdef HAVE_LIBHWLOC
|
|
bool topologyAffinity;
|
|
#endif
|
|
|
|
bool changed;
|
|
} Settings;
|
|
|
|
#define Settings_cpuId(settings, cpu) ((settings)->countCPUsFromOne ? (cpu)+1 : (cpu))
|
|
|
|
static inline ProcessField Settings_getActiveSortKey(const Settings* this) {
|
|
return (this->treeView)
|
|
? (this->treeViewAlwaysByPID ? PID : this->treeSortKey)
|
|
: this->sortKey;
|
|
}
|
|
|
|
static inline int Settings_getActiveDirection(const Settings* this) {
|
|
return this->treeView ? this->treeDirection : this->direction;
|
|
}
|
|
|
|
void Settings_delete(Settings* this);
|
|
|
|
bool Settings_write(Settings* this);
|
|
|
|
Settings* Settings_new(int initialCpuCount);
|
|
|
|
void Settings_invertSortOrder(Settings* this);
|
|
|
|
void Settings_setSortKey(Settings* this, ProcessField sortKey);
|
|
|
|
#endif
|